OPay E-Wallet Payments API
In this page, you will learn how to use E-Wallet payments with a QR code and R2P request.
- Collect your client's payment Information.
- Trigger E-Wallet payment API with your collected information.
- Handle the API Response.
- Redirect your client to scan QR code.
- After payment successfully, redirect your client to successful page.
How it works?
- Merchant will send E-Wallet Payment request with client information to OPay.
- OPay will respond to the merchant with QR code and send request to pay (R2P) to client wallet
- Merchant query order status through OPay Query Payment Status API or waiting for the callback notification
- Merchant redirect your client to the merchant successful or failure page
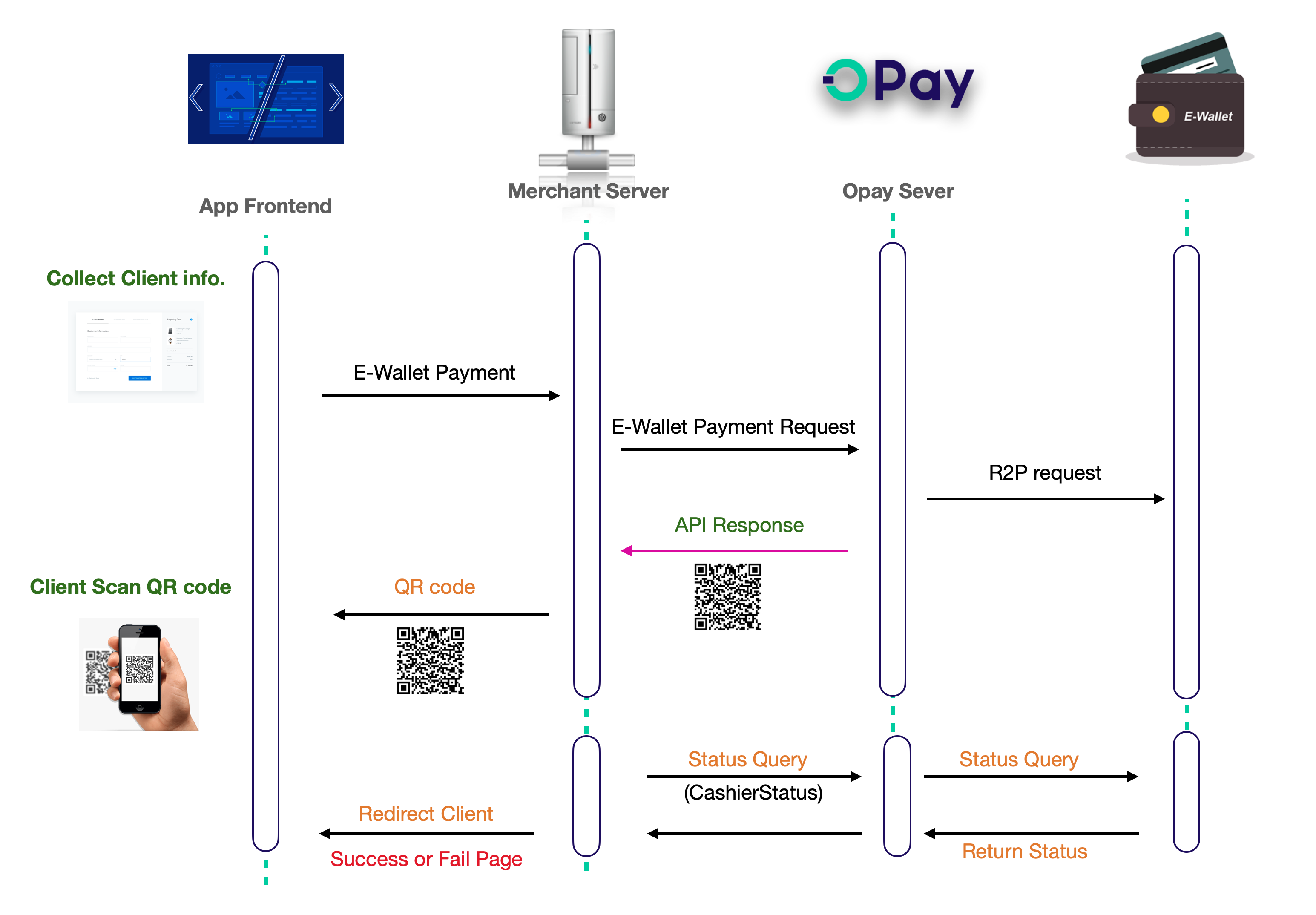
QR Code Payment
To test QR Code Payment, you need to request using the POST method in our sandbox environment.
-Here is the request URL:
https://sandboxapi.opaycheckout.com/api/v1/international/payment/create
-Once you have a fully tested payment flow and you are ready for production, use the following production API endpoint URL instead
https://api.opaycheckout.com/api/v1/international/payment/create
-Request Parameters:
- Header: Authorization(API Calls Signature), MerchantId
- Json object containing the transaction information:
Authorization : Bearer {signature}
MerchantId : 256612345678901
{
"country":"EG",
"reference":"202111300001",
"amount":{
"currency":"EGP",
"total":1000
},
"callbackUrl":"https://your-call-back-url",
"product":{
"name":"product name",
"description":"product description"
},
"userInfo":{
"userName":"OPay Test Name",
"userMobile":"201066668888",
"userEmail":"customer@email.com"
},
"userClientIP":"100.123.10.66",
"expireAt":30,
"payMethod":"MWALLET",
"walletAccount":"01066668888",
}
HTTP POST
Parameters
-Here is a detailed description for the parameters you need to complete the POST
request:
-An example of E-Wallet payment request for an amount of 400 EGP is as follows :
class MwalletController
{
private $secretkey;
private $merchantId;
private $url;
public function __construct() {
$this->merchantId = '281821110129700';
$this->secretkey = 'OPAYPRV*******0187828';
$this->url = 'https://sandboxapi.opaycheckout.com/api/v1/international/payment/create';
}
public function test(){
$data = [
'amount' => [
'currency' => 'EGP',
'total' => 400
],
'callbackUrl' => 'https://your-call-back-url.com',
'country' => 'EG',
'payMethod' => 'MWALLET',
'product' => [
'description' => 'description',
'name' => 'product-name-one'
],
'reference' => '04145678905677',
'userInfo' => [
'userEmail' => 'customer@email.com',
'userMobile' => '201066668888',
'userName' => 'OPay Test Name'
],
'walletAccount' => '01066668888'
];
$data2 = (string) json_encode($data,JSON_UNESCAPED_SLASHES);
$auth = $this->auth($data2);
$header = ['Content-Type:application/json', 'Authorization:Bearer '. $auth, 'MerchantId:'.$this->merchantId];
$response = $this->http_post($this->url, $header, json_encode($data));
$result = $response?$response:null;
return $result;
}
private function http_post ($url, $header, $data) {
if (!function_exists('curl_init')) {
throw new Exception('php not found curl', 500);
}
$ch = curl_init();
curl_setopt($ch, CURLOPT_TIMEOUT, 60);
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, false);
curl_setopt($ch, CURLOPT_HEADER, false);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $data);
curl_setopt($ch, CURLOPT_HTTPHEADER, $header);
$response = curl_exec($ch);
$httpStatusCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
$error=curl_error($ch);
curl_close($ch);
if (200 != $httpStatusCode) {
print_r("invalid httpstatus:{$httpStatusCode} ,response:$response,detail_error:" . $error, $httpStatusCode);
}
return $response;
}
public function auth ( $data ) {
$secretKey = $this->secretkey;
$auth = hash_hmac('sha512', $data, $secretKey);
return $auth;
}
}
# importing the requests library
import requests
# API Endpoint
URL = "https://sandboxapi.opaycheckout.com/api/v1/international/payment/create"
# defining a params dict for the parameters to be sent to the API
PaymentData = {
'reference' : '12312321324',
'amount': {
"total": '400',
"currency": 'EGP',
},
'product': {
"name": 'Iphone X',
"description": 'xxxxxxxxxxxxxxxxx',
},
'callbackUrl' : 'your callbackUrl',
'country' : 'EG',
'expireAt' : ':30',
'payMethod' : 'MWALLET',
'walletAccount':'01066668888',
'userInfo': {
"userName": 'your customer name',
"userMobile": '201312312xxx',
"userEmail": 'xxx@xxx.com',
},
}
# sending post request and saving the response as response object
status_request = requests.post(url = URL, params = json.dumps(PaymentData))
# extracting data in json format
status_response = status_request.json()
const request = require('request');
var sha512 = require('js-sha512');
const formData = {
"amount":{
"currency":"EGP",
"total":400
},
"callbackUrl":"https://your-call-back-url.com",
"country":"EG",
"payMethod":"MWALLET",
"product":{
"description":"description",
"name":"product-name-one"
},
"reference":"04122345678964",
"userInfo":{
"userEmail":"customer@email.com",
"userMobile":"201066668888",
"userName":"OPay Test Name"
},
"walletAccount":"01066668888"
};
var privateKey = "OPAYPRV*******0187828"
var hash = sha512.hmac.create(privateKey);
hash.update(JSON.stringify(formData));
hmacsignature = hash.hex();
console.log(hmacsignature)
request({
url: 'https://sandboxapi.opaycheckout.com/api/v1/international/payment/create',
method: 'POST',
headers: {
'MerchantId': '256621051120756',
'Authorization': 'Bearer '+hmacsignature
},
json: true,
body: formData
}, function (error, response, body) {
console.log('body: ')
console.log(body)
}
)
curl --location --request POST 'https://sandboxapi.opaycheckout.com/api/v1/international/payment/create' \
--header 'MerchantId: 256621051120756' \
--header 'Authorization: Bearer 38017496e*******2ab0297d1de8905cb7d85a043d9084254e61a8da6093b6f155550ff51891dca9f9889a' \
--header 'Content-Type: application/json' \
--data-raw '{
"amount":{
"currency":"EGP",
"total":400
},
"callbackUrl":"https://your-call-back-url.com",
"country":"EG",
"payMethod":"MWALLET",
"product":{
"description":"description",
"name":"name"
},
"reference":"041233981111",
"userInfo":{
"userEmail":"customer@email.com",
"userMobile":"201066668888",
"userName":"OPay Test Name"
},
"walletAccount":"01066668888"
}'
import com.google.gson.Gson;
import org.apache.commons.codec.binary.Hex;
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import java.nio.charset.StandardCharsets;
import java.util.TreeMap;
import java.util.UUID;
public class Mwallet {
private static final String privateKey = "OPAYPRV*******0187828";
private static final String endpoint = "https://sandboxapi.opaycheckout.com";
private static final String merchantId = "256621051120756";
public static void main(String[] args) throws Exception {
String addr = endpoint + "/api/v1/international/payment/create";
Gson gson = new Gson();
TreeMap order = new TreeMap<>();
TreeMap amount = new TreeMap<>();
amount.put("currency","EGP");
amount.put("total",new Integer(400));
order.put("amount",amount);
order.put("callbackUrl","https://your-call-back-url.com");
order.put("country","EG");
order.put("payMethod","MWALLET");
TreeMap product = new TreeMap<>();
product.put("description","description");
product.put("name","name");
order.put("product",product);
order.put("reference", UUID.randomUUID().toString());
TreeMap userInfo = new TreeMap<>();
userInfo.put("userEmail","customer@email.com");
userInfo.put("userMobile","201066668888");
userInfo.put("userName","OPay Test Name");
order.put("userInfo",userInfo);
order.put("walletAccount","01066668888");
String requestBody = gson.toJson(order);
System.out.println("--request:");
System.out.println(requestBody);
String oPaySignature = hmacSHA512(requestBody, privateKey);
System.out.println("--signature:");
System.out.println(oPaySignature);
URL url = new URL(addr);
HttpURLConnection con = (HttpURLConnection)url.openConnection();
con.setRequestMethod("POST");
con.setRequestProperty("Content-Type", "application/json; utf-8");
con.setRequestProperty("Authorization", "Bearer "+oPaySignature);
con.setRequestProperty("MerchantId", merchantId);
con.setDoOutput(true);
OutputStream os = con.getOutputStream();
byte[] input = requestBody.getBytes(StandardCharsets.UTF_8);
os.write(input, 0, input.length);
BufferedReader br = new BufferedReader(new InputStreamReader(con.getInputStream(), StandardCharsets.UTF_8));
StringBuilder response = new StringBuilder();
String responseLine = null;
while ((responseLine = br.readLine()) != null) {
response.append(responseLine.trim());
}
System.out.println("--response:");
System.out.println(response.toString());
//close your stream and connection
}
public static String hmacSHA512(final String data, final String secureKey) throws Exception{
byte[] bytesKey = secureKey.getBytes();
final SecretKeySpec secretKey = new SecretKeySpec(bytesKey, "HmacSHA512");
Mac mac = Mac.getInstance("HmacSHA512");
mac.init(secretKey);
final byte[] macData = mac.doFinal(data.getBytes());
byte[] hex = new Hex().encode(macData);
return new String(hex, StandardCharsets.UTF_8);
}
}
using System;
using System.IO;
using System.Linq;
using System.Net;
using System.Text;
using Newtonsoft.Json;
namespace OPayRequest
{
public class Program
{
static void Main(string[] args)
{
PostJson("https://sandboxapi.opaycheckout.com/api/v1/international/payment/create", new opay_request
{
reference = '12312321324',
amount= {
"total"= '400',
"currency"= 'EGP',
},
product= {
"name"= 'Iphone X',
"description"= 'xxxxxxxxxxxxxxxxx',
},
callbackUrl = 'your callbackUrl',
country = 'EG';
expireAt = ':30';
merchantName = 'your merchant name';
payMethod = 'MWALLET';
walletAccount = '01066668888';
userInfo = {
"userName": 'your customer name',
"userMobile": '201312312xxx',
"userEmail": 'xxx@xxx.com',
},
});
}
private static void PostJson(string uri, opay_request postParameters)
{
string postData = JsonConvert.SerializeObject(postParameters);
byte[] bytes = Encoding.UTF8.GetBytes(postData);
var httpWebRequest = (HttpWebRequest) WebRequest.Create(uri);
httpWebRequest.Method = "POST";
httpWebRequest.ContentLength = bytes.Length;
httpWebRequest.ContentType = "text/json";
using (Stream requestStream = httpWebRequest.GetRequestStream())
{
requestStream.Write(bytes, 0, bytes.Count());
}
var httpWebResponse = (HttpWebResponse) httpWebRequest.GetResponse();
if (httpWebResponse.StatusCode != HttpStatusCode.OK)
{
string message = String.Format("GET failed. Received HTTP {0}", httpWebResponse.StatusCode);
throw new ApplicationException(message);
}
}
}
public class opay_request
{
public string reference { get; set; }
public string callbackUrl { get; set; }
public string expireAt { get; set; }
public string country { get; set; }
public string expireAt { get; set; }
public string merchantName { get; set; }
public string payMethod { get; set; }
public string walletAccount { get; set; }
public product product;
public amount amount;
public userInfo userInfo;
}
public class amount
{
public string total { get; set; }
public string currency { get; set; }
}
public class product
{
public string name { get; set; }
public string description { get; set; }
}
public class userInfo
{
public string userName { get; set; }
public string userEmail { get; set; }
public string userMobile { get; set; }
}
}
E-Wallet Payment Response
-Response Parameters:
the parameters contained in the response received whenever you call the E-Wallet payment API as a JSON Object.
{
"code": "00000",
"message": "SUCCESSFUL",
"data": {
"reference": "202111300001",
"orderNo": "211130144654879720",
"nextAction": {
"actionType": "SCAN_QR_CODE",
"qrCode":"data:image/png;base64,iVBORw0KGgoAAAANS…="
},
"status": "PENDING",
"amount": {
"total": 0,
"currency": "EGP"
},
"vat": {
"total": 0,
"currency": "EGP"
}
}
}
-Here is a detailed description for the parameters received in the response:
Parameter | type | Description | example | |
---|---|---|---|---|
reference | String |
The unique merchant payment order number. | 202111300001 | |
orderNo | String |
OPay transaction number | 211130148131809099 | |
nextAction
Json Object
|
||||
actionType | String |
The next action type.Waiting for customer scan QR code | SCAN_QR_CODE | |
qrCode | String |
The QR code formatted in ISO. | "0002010102120216…" | |
status | String |
E-Wallet order status | PENDING | |
amount
Json Object
|
||||
total | Long |
Transaction amount | 1000 (cent unit) | |
currency | String |
currency type. | EGP | |
vat
Json Object
|
||||
total | String |
Value Added Tax Amount | 0 | |
currency | String |
currency type. | EGP |
Error Handling
After submitting an API call to OPay, you receive a response back to inform you that
your request was received and processed. A successful OPay API should return a status code
00
,
meanwhile, in a situation where any payment processing error occurred, you will receive an error code
with a
message to describe the reason of the error. A sample error response can be found below.
{
"code": "20000",
"message": "Duplicate merchant order number",
"data": null
}
Depending on the HTTP status code of the response, you should build some logic to handle any errors that a request or the system may return. A list of possible potential error codes that you may receive can be found below. A full list of all possible error codes can be found in the Error Codes section.
Error Code | Error Message |
---|---|
02000 | Time out. |
02001 | authentication failed. |
50003 | request parameters not valid. |